C# Windows Media Player Audio/Video Play #2
C# 2021. 11. 21. 17:20 |반응형
C#에서 Windows Media Player를 이용해 오디오 파일을 플레이 해 보자.
아래 링크를 참고해 Windows Media Player COM Component를 가져오고 빌드시 메세지가 발생하지 않도록 하자.
(Windows Media Player 컴포넌트를 폼에 배치할 필요는 없다)
2021.11.21 - [C#] - C# Windows Media Player Audio/Video Play #1
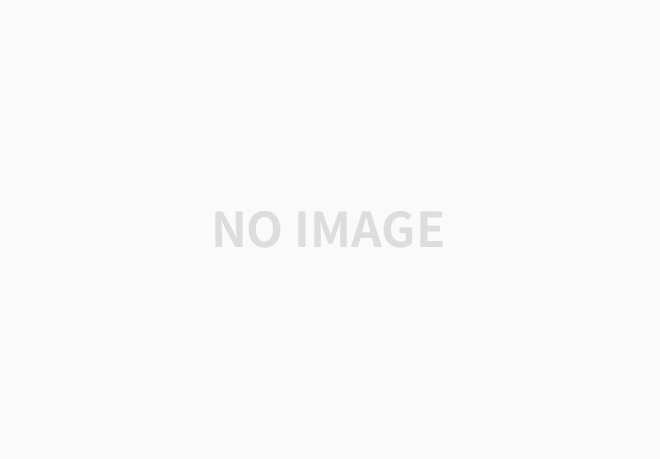
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
|
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
using WMPLib;
namespace WindowsFormsApp1
{
public partial class Form1 : Form
{
private string time;
WindowsMediaPlayer wmp;
public Form1()
{
InitializeComponent();
wmp = new WindowsMediaPlayer();
Timer T = new Timer();
T.Interval = 1000;
T.Tick += new EventHandler(Form1_Timer);
T.Start();
}
private void Form1_Timer(object sender, System.EventArgs e)
{
if (wmp.playState == WMPPlayState.wmppsPlaying)
{
//time = wmp.controls.currentPositionString + " / " + wmp.controls.currentItem.durationString;
//time = wmp.controls.currentPosition.ToString() + " / " + wmp.controls.currentItem.duration.ToString();
time = TimeSpan.FromSeconds((int)wmp.controls.currentPosition).ToString() + " / "
+ TimeSpan.FromSeconds((int)wmp.controls.currentItem.duration);
Graphics G = CreateGraphics();
//G.DrawString(time, Font, System.Drawing.Brushes.Black, 20, 70); // 글자가 겹친다.
TextRenderer.DrawText(G, time, Font, new Point(20, 70), ForeColor, BackColor);
G.Dispose();
}
}
private void button1_Click(object sender, EventArgs e)
{
try
{
OpenFileDialog dlg = new OpenFileDialog();
if (dlg.ShowDialog() == DialogResult.OK)
{
wmp.URL = dlg.FileName;
}
}
catch (Exception exc)
{
MessageBox.Show(exc.Message);
}
}
private void button2_Click(object sender, EventArgs e)
{
if (wmp.playState == WMPPlayState.wmppsPlaying)
{
wmp.controls.stop();
}
}
}
}
|
소스를 입력한다.
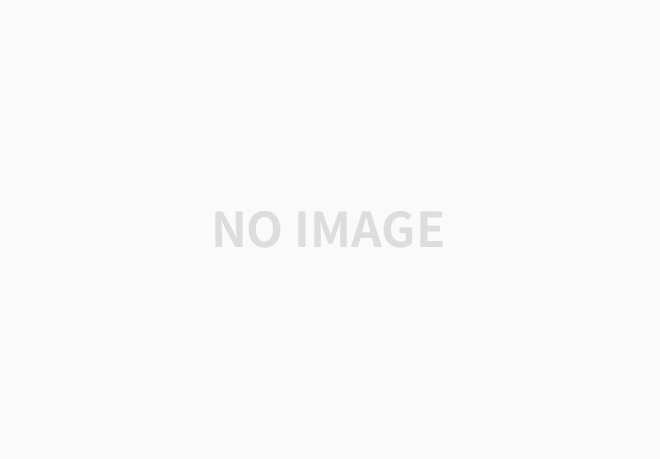
실행 파일과 함께 Interop.WMPLib.dll 파일이 존재해야 한다.
Using the Windows Media Player Control in a .NET Framework Solution
반응형
'C#' 카테고리의 다른 글
C# Screen Capture - 스크린 캡쳐 (0) | 2021.11.22 |
---|---|
C# Form Designer Layout Mode - 폼 디자이너 레이아웃 모드 (0) | 2021.11.22 |
C# Windows Media Player Audio/Video Play #1 (0) | 2021.11.21 |
C# Media Player Mp3 (0) | 2021.11.21 |
C# I Love You Program - 사랑해 프로그램 (0) | 2021.11.20 |