C# Joystick(Gamepad) Input Check - 조이스틱(게임패드) 입력 확인 1
C# 2022. 4. 5. 17:02 |컴퓨터에 연결된 모든 HID(Human Interface Devices)를 확인하고 조이스틱(게임패드)의 입력을 체크해 보자.
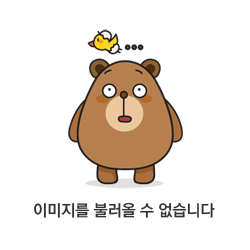
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
130
131
132
133
134
135
136
137
|
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Threading;
using System.Diagnostics;
using HidSharp;
using HidSharp.Utility;
using HidSharp.Reports;
namespace ConsoleApp1
{
class Program
{
static void WriteDeviceItemInputParserResult(HidSharp.Reports.Input.DeviceItemInputParser parser)
{
while (parser.HasChanged)
{
int changedIndex = parser.GetNextChangedIndex();
DataValue previousDataValue = parser.GetPreviousValue(changedIndex);
DataValue dataValue = parser.GetValue(changedIndex);
Console.WriteLine(string.Format(" {0}: {1} -> {2}", (Usage)dataValue.Usages.FirstOrDefault(),
previousDataValue.GetPhysicalValue(), dataValue.GetPhysicalValue()));
}
}
static void Main(string[] args)
{
HidSharpDiagnostics.EnableTracing = true;
HidSharpDiagnostics.PerformStrictChecks = true;
DeviceList list = DeviceList.Local;
list.Changed += (sender, e) => Console.WriteLine("Device list changed.");
Device[] allDeviceList = list.GetAllDevices().ToArray();
Console.WriteLine("■ All device list:");
foreach (Device dev in allDeviceList)
{
Console.WriteLine("- Name: " + dev.ToString() + "\n" + " Path: " + dev.DevicePath);
}
Console.WriteLine("-----------------------------------------------");
Stopwatch stopwatch = Stopwatch.StartNew();
HidDevice[] hidDeviceList = list.GetHidDevices().ToArray();
Console.WriteLine("■ HID device list (took {0} ms to get {1} devices):",
stopwatch.ElapsedMilliseconds, hidDeviceList.Length);
foreach (HidDevice dev in hidDeviceList)
{
Console.WriteLine("- Name: " + dev);
Console.WriteLine(" Path: " + dev.DevicePath);
try
{
ReportDescriptor reportDescriptor = dev.GetReportDescriptor();
// Lengths should match.
Debug.Assert(dev.GetMaxInputReportLength() == reportDescriptor.MaxInputReportLength);
Debug.Assert(dev.GetMaxOutputReportLength() == reportDescriptor.MaxOutputReportLength);
Debug.Assert(dev.GetMaxFeatureReportLength() == reportDescriptor.MaxFeatureReportLength);
foreach (DeviceItem deviceItem in reportDescriptor.DeviceItems)
{
foreach (uint usage in deviceItem.Usages.GetAllValues())
{
Console.WriteLine(string.Format(" Usage: {0:X4} {1}", usage, (Usage)usage));
}
{
Console.WriteLine("Opening device for 20 seconds...");
HidStream hidStream;
if (dev.TryOpen(out hidStream))
{
Console.WriteLine("Opened device.");
hidStream.ReadTimeout = Timeout.Infinite;
using (hidStream)
{
byte[] inputReportBuffer = new byte[dev.GetMaxInputReportLength()];
HidSharp.Reports.Input.HidDeviceInputReceiver inputReceiver =
reportDescriptor.CreateHidDeviceInputReceiver();
HidSharp.Reports.Input.DeviceItemInputParser inputParser =
deviceItem.CreateDeviceItemInputParser();
inputReceiver.Start(hidStream);
int startTime = Environment.TickCount;
while (true)
{
if (inputReceiver.WaitHandle.WaitOne(1000))
{
if (!inputReceiver.IsRunning) { break; } // Disconnected?
Report report;
while (inputReceiver.TryRead(inputReportBuffer, 0, out report))
{
// Parse the report if possible.
// This will return false if (for example) the report applies to a different DeviceItem.
if (inputParser.TryParseReport(inputReportBuffer, 0, report))
{
WriteDeviceItemInputParserResult(inputParser);
}
}
}
uint elapsedTime = (uint)(Environment.TickCount - startTime);
if (elapsedTime >= 20000) { break; } // Stay open for 20 seconds.
}
}
Console.WriteLine("Closed device.");
}
else
{
Console.WriteLine("Failed to open device.");
}
Console.WriteLine(" ---------------------------------------------");
}
}
}
catch (Exception e)
{
Console.WriteLine(e);
}
}
}
}
}
|
소스를 입력하고 빌드한다.
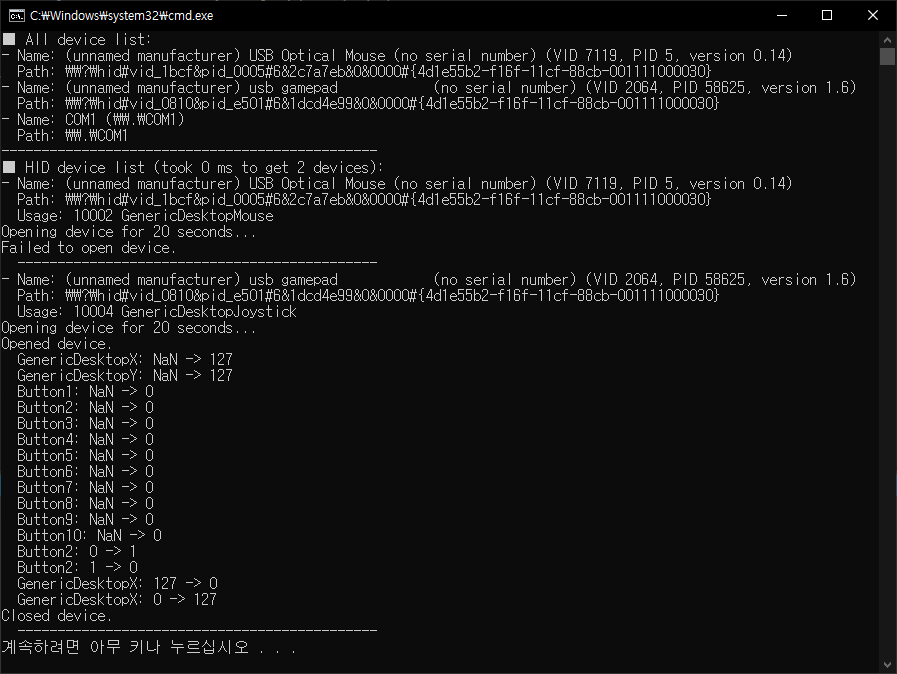
포커스가 다른 프로그램에 있어도 디바이스의 입력을 확인 할 수 있다.
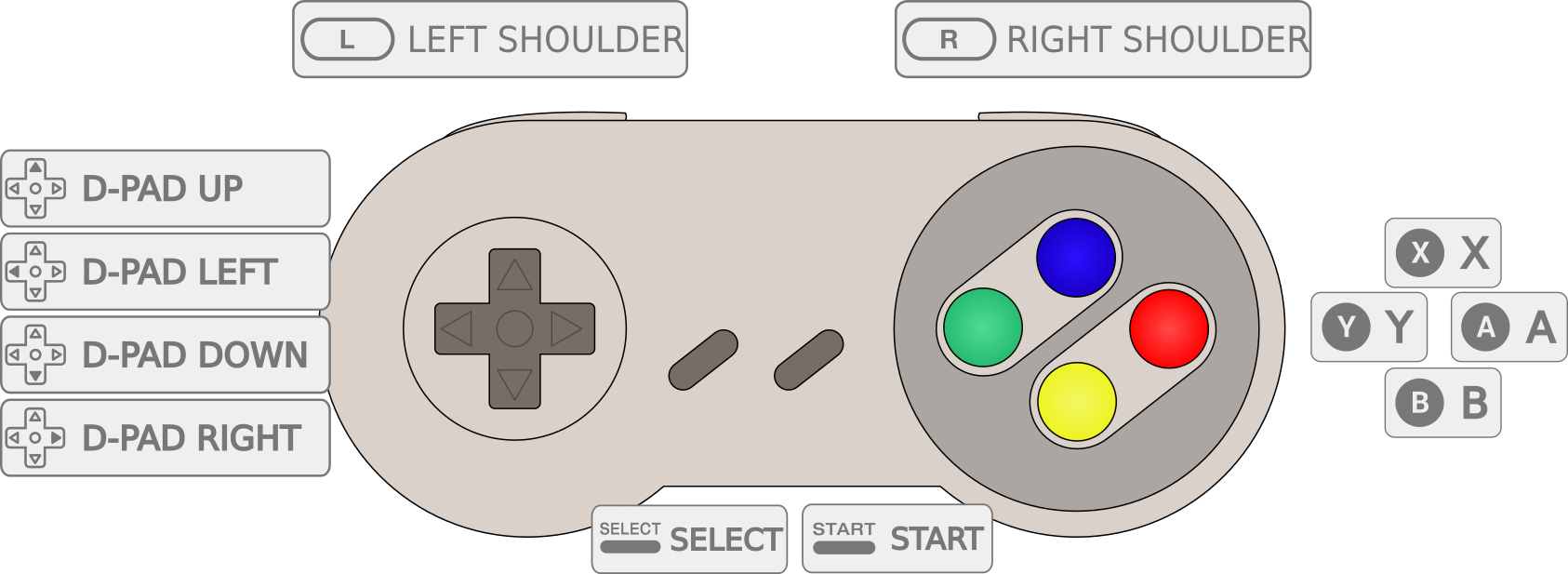
각 버튼이 눌렸을때 inputReportBuffer의 변화되는 내용을 확인해 보면 아래와 같다.
(첫 번째 바이트의 '01'은 Report ID인거 같다)
1
|
Console.WriteLine(BitConverter.ToString(inputReportBuffer));
|
01-80-80-7F-7F-0F-00-00
01-80-80-00-7F-0F-00-00
GenericDesktopX: 127 -> 0
01-80-80-7F-7F-0F-00-00
01-80-80-FF-7F-0F-00-00
GenericDesktopX: 127 -> 255
01-80-80-7F-7F-0F-00-00
01-80-80-7F-00-0F-00-00
GenericDesktopY: 127 -> 0
01-80-80-7F-7F-0F-00-00
01-80-80-7F-FF-0F-00-00
GenericDesktopY: 127 -> 255
※게임패드의 방향버튼은 기본이(버튼이 눌리지 않았을때) 127
01-80-80-7F-7F-0F-00-00
01-80-80-7F-7F-1F-00-00
Button1: 0 -> 1
01-80-80-7F-7F-0F-00-00
01-80-80-7F-7F-2F-00-00
Button2: 0 -> 1
01-80-80-7F-7F-0F-00-00
01-80-80-7F-7F-4F-00-00
Button3: 0 -> 1
01-80-80-7F-7F-0F-00-00
01-80-80-7F-7F-8F-00-00
Button4: 0 -> 1
※게임패드의 버튼은 기본이(버튼이 눌리지 않았을때) 0
'C#' 카테고리의 다른 글
C# Type Class 타입 클래스 (0) | 2022.07.20 |
---|---|
C# Joystick(Gamepad) Input Check - 조이스틱(게임패드) 입력 확인 2 (0) | 2022.04.06 |
C# Console Key Input Check - 콘솔 키 입력 확인 (0) | 2022.04.05 |
C# Drag & Drop - 드래그 & 드롭 2 (0) | 2022.03.31 |
C# Drag & Drop - 드래그 & 드롭 1 (0) | 2022.03.31 |