Execute Adb Shell Command 안드로이드 리눅스 커널 명령 실행
Android 2022. 4. 20. 13:51 |반응형
안드로이드 리눅스 커널 명령을 실행해 보자.
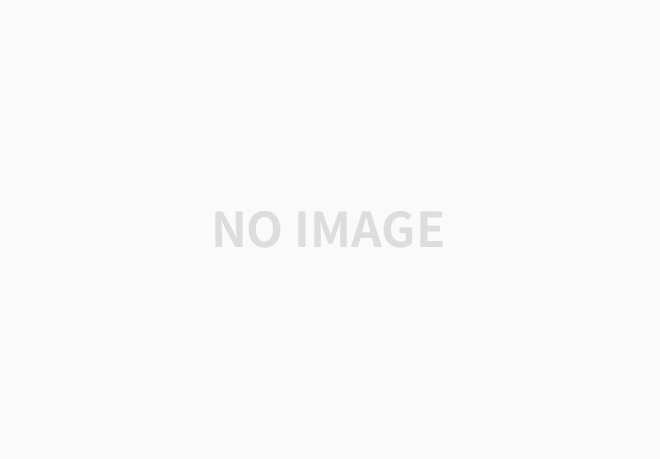
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
|
package com.example.myapplication;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.TextView;
import java.io.BufferedReader;
import java.io.InputStreamReader;
public class MainActivity extends AppCompatActivity {
EditText editText;
Button button;
TextView textView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
editText = findViewById(R.id.editTextTextPersonName);
button = findViewById(R.id.button);
textView = findViewById(R.id.textView);
button.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
StringBuffer stringBuffer = new StringBuffer();
java.lang.Process process;
try {
process = Runtime.getRuntime().exec(editText.getText().toString());
process.waitFor();
// Causes the current thread to wait, if necessary, until the process
// represented by this Process object has terminated.
BufferedReader bufferedReader = new BufferedReader(new InputStreamReader(process.getInputStream()));
String line = "";
while ((line = bufferedReader.readLine()) != null) {
stringBuffer.append(line + "\n");
}
} catch (Exception e) {
e.printStackTrace();
}
String result = stringBuffer.toString();
textView.setText(result);
}
});
}
}
|
소스를 입력하고 빌드한다.
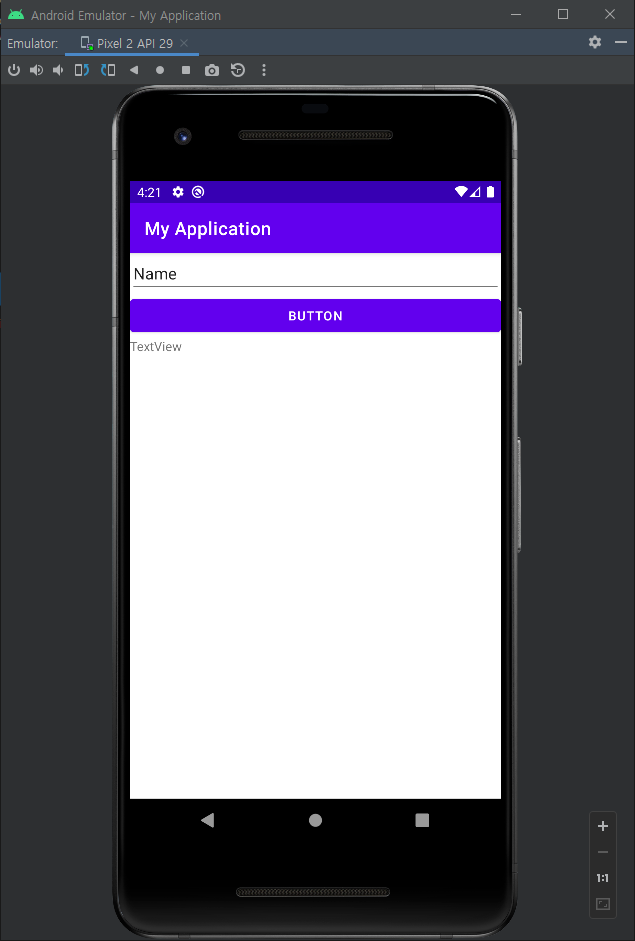
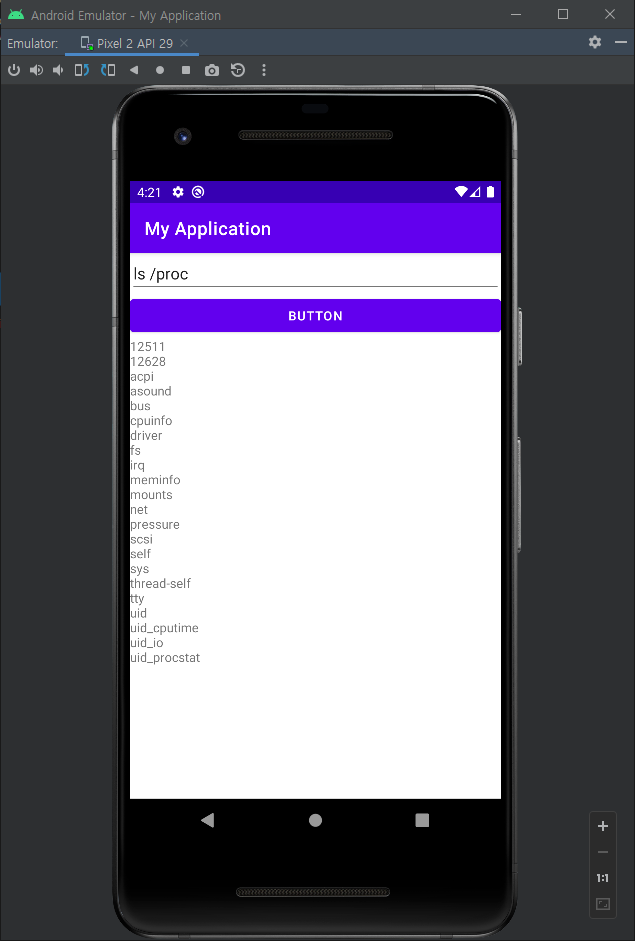

예) cd /proc, pwd를 차례로 실행해도 결과는 항상 /이다.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
|
package com.example.myapplication;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.TextView;
public class MainActivity extends AppCompatActivity {
EditText editText;
Button button;
TextView textView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
editText = findViewById(R.id.editTextTextPersonName);
button = findViewById(R.id.button);
textView = findViewById(R.id.textView);
button.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
try {
Runtime.getRuntime().exec(editText.getText().toString());
} catch (Exception e) {
e.printStackTrace();
}
}
});
}
}
|
소스를 위와 같이 바꾸고 input 명령어를 사용해 보자.
(process.waitFor() 를 사용하면 아래 예에서 제대로 동작하지 않는다)
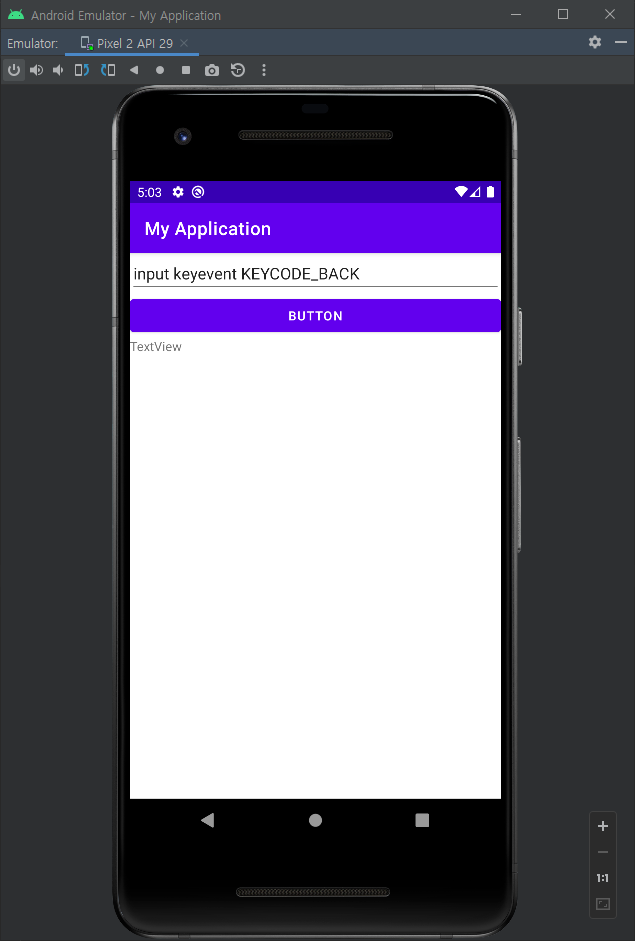
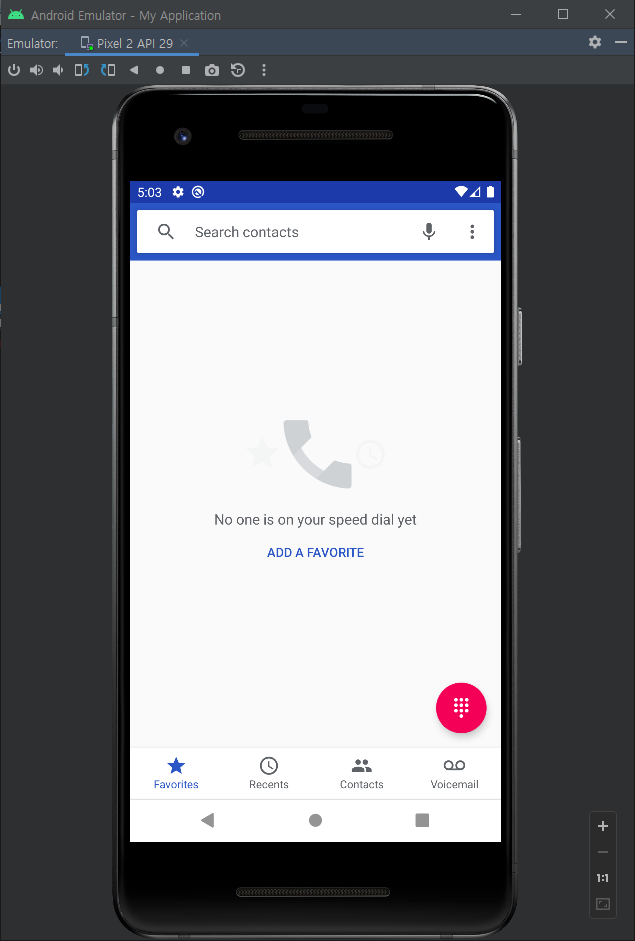
※ 참고
반응형
'Android' 카테고리의 다른 글
ViewPager2 뷰페이저2 (0) | 2023.07.11 |
---|---|
RecyclerView 리사이클러뷰 (0) | 2023.07.11 |
Text To Speech 안드로이드 TTS (0) | 2022.02.14 |
Message Passing with Thread 메인 스레드에서 다른 스레드로 메세지(데이터) 보내기 (0) | 2022.02.14 |
User Interface Manipulation From UI Thread - 다른 스레드에서 UI 객체 접근하기 (0) | 2022.02.13 |