Python C API
C, C++ 2018. 11. 21. 15:52 |반응형
가장 간단하게 Python을 C에 embed 시키는 방법은 Very High Level interface를 사용 하는 것이다. VHL interface는 어플리케이션과 직접적인 상관 없이 Python script를 실행하기 위한 것이다.
관련 문서
우선 Python C API 관련 Include, Library 디렉토리를 프로젝트에 추가한다.
C:\Users\UserName\AppData\Local\Programs\Python\PythonXXX\include
C:\Users\UserName\AppData\Local\Programs\Python\PythonXXX\libs
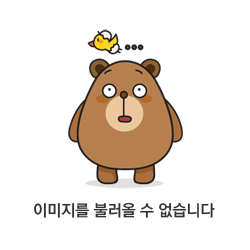
파이썬 공식 홈페이지에서 다운 받은 파이썬 인스톨러 패키지를 설치 했다면 Solution Configurations를 Release로 설정 한다. Debug 모드로 build 할 경우 pythonXX_d.lib를 찾을 수 없다는 에러가 발생한다. pythonXX_d.lib 파일을 생성하려면 파이썬 소스를 받아서 직접 컴파일해야 한다.
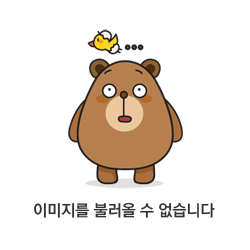
예제:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
|
#pragma comment(lib, "python36.lib")
#include <Python.h>
int main(int argc, char *argv[])
{
wchar_t *program = Py_DecodeLocale(argv[0], NULL);
// Decode a byte string from the locale encoding with the surrogateescape error handler:
// undecodable bytes are decoded as characters in range U+DC80..U+DCFF. If a byte sequence
// can be decoded as a surrogate character, escape the bytes using the surrogateescape error
// handler instead of decoding them.
// Return a pointer to a newly allocated wide character string, use PyMem_RawFree() to free
// the memory. If size is not NULL, write the number of wide characters excluding the null
// character into *size
if (program == NULL) {
fprintf(stderr, "Fatal error: cannot decode argv[0]\n");
exit(1);
}
Py_SetProgramName(program); /* optional but recommended */
// This function should be called before Py_Initialize() is called for the first time, if it
// is called at all.It tells the interpreter the value of the argv[0] argument to the main()
// function of the program(converted to wide characters).This is used by Py_GetPath() and some
// other functions below to find the Python run - time libraries relative to the interpreter
// executable.
Py_Initialize();
// Initialize the Python interpreter. In an application embedding Python, this should be called
// before using any other Python/C API functions
if (Py_IsInitialized())
// Return true (nonzero) when the Python interpreter has been initialized, false (zero) if not.
// After Py_FinalizeEx() is called, this returns false until Py_Initialize() is called again.
{
PyRun_SimpleString("print('Hello Python')");
// This is a simplified interface to PyRun_SimpleStringFlags(), leaving the PyCompilerFlags*
// argument set to NULL.
PyRun_SimpleString("from time import time, ctime\n"
"print('Today is', ctime(time()))\n");
//PyRun_SimpleString("from time import time, ctime\nprint('Today is', ctime(time()))\n");
if (Py_FinalizeEx() < 0)
// Undo all initializations made by Py_Initialize() and subsequent use of Python/C API
// functions, and destroy all sub-interpreters that were created and not yet destroyed
// since the last call to Py_Initialize().
{
exit(120);
}
PyMem_RawFree(program);
// Frees the memory block pointed to by p, which must have been returned by a previous call
// to PyMem_RawMalloc(), PyMem_RawRealloc() or PyMem_RawCalloc().
// Otherwise, or if PyMem_RawFree(p) has been called before, undefined behavior occurs.
// If p is NULL, no operation is performed.
}
return 0;
}
|
cs |
반응형
'C, C++' 카테고리의 다른 글
Qt 스프라이트 애니매이션 (0) | 2019.02.21 |
---|---|
Qt 설치 및 간단한 사용 예 (4) | 2019.01.16 |
How to install and use JsonCpp - JsonCpp 설치 및 사용 (2) | 2019.01.08 |
Console 어플리케이션에 이미지 디스플레이 하기 (0) | 2018.11.22 |
MySQL C API (0) | 2018.11.20 |