C# AudioSwitcher System Audio/Sound Volume Control - 시스템 오디오/사운드 볼륨 컨트롤 2
C# 2022. 1. 7. 16:26 |반응형
2022.01.06 - [C#] - C# AudioSwitcher System Audio/Sound Volume Control - 시스템 오디오/사운드 볼륨 컨트롤 1
의 소스를 수정해 Observer를 등록하고 VolumeChanged Notification을 받아 지정된 볼륨 이상 변경 하지 못 하도록 해 보자.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
|
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Runtime.InteropServices;
using AudioSwitcher.AudioApi.CoreAudio;
using AudioSwitcher.AudioApi;
namespace ConsoleApp1
{
class Program
{
[DllImport("kernel32.dll")]
static extern IntPtr GetConsoleWindow();
[DllImport("user32.dll")]
static extern bool ShowWindow(IntPtr hWnd, int nCmdShow);
static int maxVol;
static void Main(string[] args)
{
const int SW_HIDE = 0; // 창 숨기기
const int SW_SHOW = 1; // 창 보이기
IntPtr handle = GetConsoleWindow();
//ShowWindow(handle, SW_HIDE);
CoreAudioDevice defaultPlaybackDevice = new CoreAudioController().DefaultPlaybackDevice;
Console.WriteLine($"Initial Volume: {defaultPlaybackDevice.Volume}");
IObserver<DeviceVolumeChangedArgs> volumeChangeObserver = new VolumeChangeObserver();
IDisposable subscriber = defaultPlaybackDevice.VolumeChanged.Subscribe(volumeChangeObserver);
//subscriber.Dispose(); // 볼륨 제한 종료
if (args.Length > 0)
{
maxVol = int.Parse(args[0]);
}
else
{
maxVol = 30;
}
defaultPlaybackDevice.Volume = maxVol; // 허용 최고 볼륨으로 초기화
while (true)
{
System.Threading.Thread.Sleep(10000); // 10초 지연
}
}
public class VolumeChangeObserver : IObserver<DeviceVolumeChangedArgs>
{
public virtual void OnCompleted()
{
Console.WriteLine("Completed.");
}
public virtual void OnError(Exception e)
{
Console.WriteLine(e.Message);
}
public virtual void OnNext(DeviceVolumeChangedArgs args)
{
if (args.Volume > maxVol)
{
Console.WriteLine($"Volume limit: {maxVol}");
args.Device.Volume = maxVol; // args.Volume = read only
}
else
{
Console.WriteLine($"Current volume: {args.Volume}");
}
}
}
}
}
|
소스를 입력하고 빌드한다.
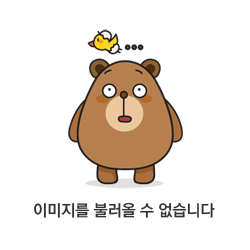
※ 참고
2022.01.08 - [C#] - C# Observer Design Pattern with The IObserver and IObservable interfaces
2023.10.26 - [Python] - Python Core Audio Windows Library 파이썬 코어 오디오 라이브러리
2023.10.28 - [C#] - C# Sound Meter 사운드 미터
반응형