[Godot] QueueFree() 노드 삭제
Godot 2023. 9. 20. 12:26 |반응형
추가한 캐릭터를 삭제해 보자.
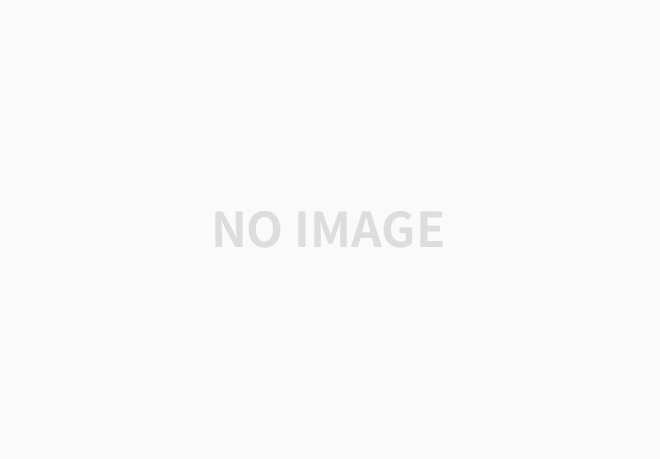
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
|
using Godot;
public partial class Character : Sprite2D
{
// Called when the node enters the scene tree for the first time.
public override void _Ready()
{
}
// Called every frame. 'delta' is the elapsed time since the previous frame.
public override async void _Process(double delta)
{
await ToSignal(GetTree().CreateTimer(1.0), SceneTreeTimer.SignalName.Timeout);
QueueFree();
}
}
|
1초 대기 후 큐에서 삭제하는 스크립트를 작성한다.
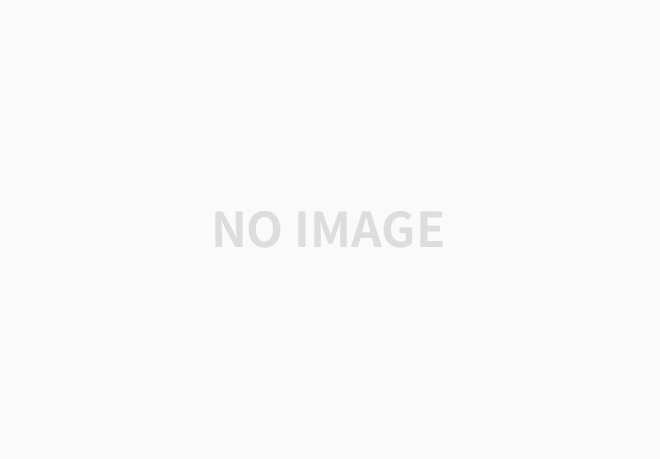
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
|
using Godot;
public partial class Main : Node
{
[Export]
public PackedScene CharacterScene { get; set; }
// Called when the node enters the scene tree for the first time.
public override void _Ready()
{
}
// Called every frame. 'delta' is the elapsed time since the previous frame.
public override void _Process(double delta)
{
if (Input.IsActionJustPressed("lclick"))
{
Character character = CharacterScene.Instantiate<Character>();
character.Position = GetViewport().GetMousePosition();
AddChild(character);
}
}
}
|
왼쪽 마우스 버튼을 클릭하면 캐릭터를 생성하는 스크립트를 작성한다.
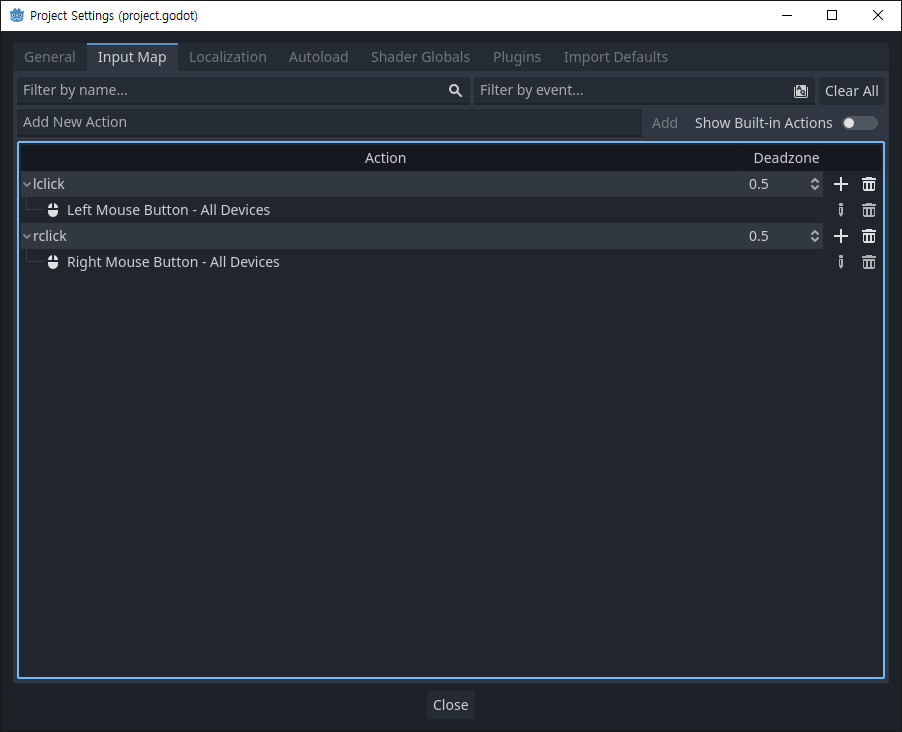
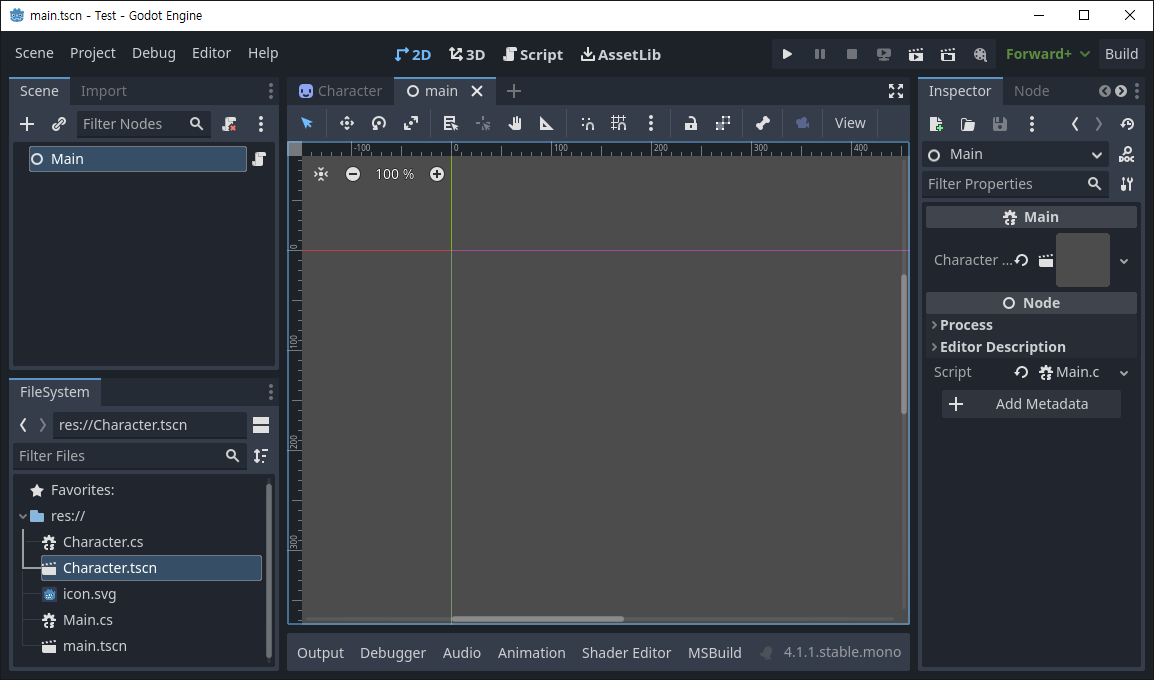

이번엔 특정 액션(마우스 오른쪽 버튼 클릭) 발생시 모든 캐릭터를 한번에 삭제해 보자.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
|
using Godot;
public partial class Character : Sprite2D
{
// Called when the node enters the scene tree for the first time.
public override void _Ready()
{
}
// Called every frame. 'delta' is the elapsed time since the previous frame.
public override async void _Process(double delta)
{
//await ToSignal(GetTree().CreateTimer(1.0), SceneTreeTimer.SignalName.Timeout);
//QueueFree();
}
}
|
캐릭터 클래스에서 1초 대기 후 큐에서 삭제하는 코드를 삭제한다.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
|
using Godot;
public partial class Main : Node
{
[Export]
public PackedScene CharacterScene { get; set; }
// Called when the node enters the scene tree for the first time.
public override void _Ready()
{
}
// Called every frame. 'delta' is the elapsed time since the previous frame.
public override void _Process(double delta)
{
if (Input.IsActionJustPressed("lclick"))
{
Character character = CharacterScene.Instantiate<Character>();
character.Position = GetViewport().GetMousePosition();
AddChild(character);
}
if (Input.IsActionJustPressed("rclick"))
{
GetTree().CallGroup("chargroup", Node.MethodName.QueueFree);
}
}
}
|
메인 클래스에서 마우스 우클릭시 "chargroup"에 속한 노드의 QueueFree()를 호출하는 코드를 작성한다.
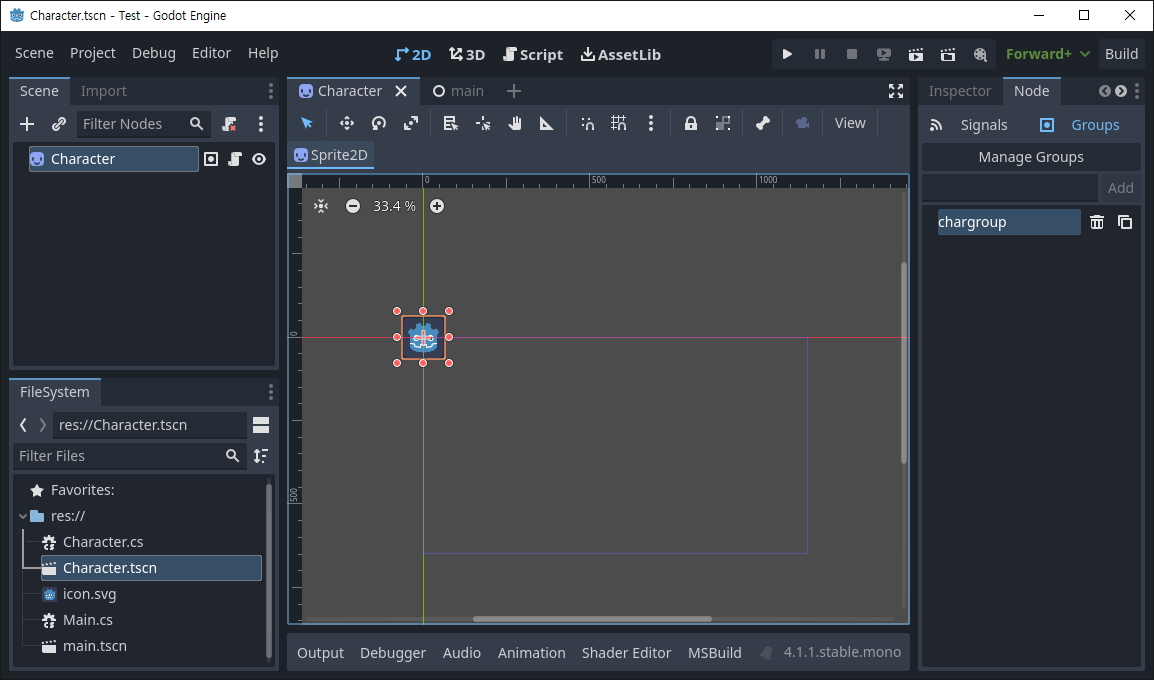
Godot의 group은 다른 게임엔진의 tag와 같은 역할을 한다.
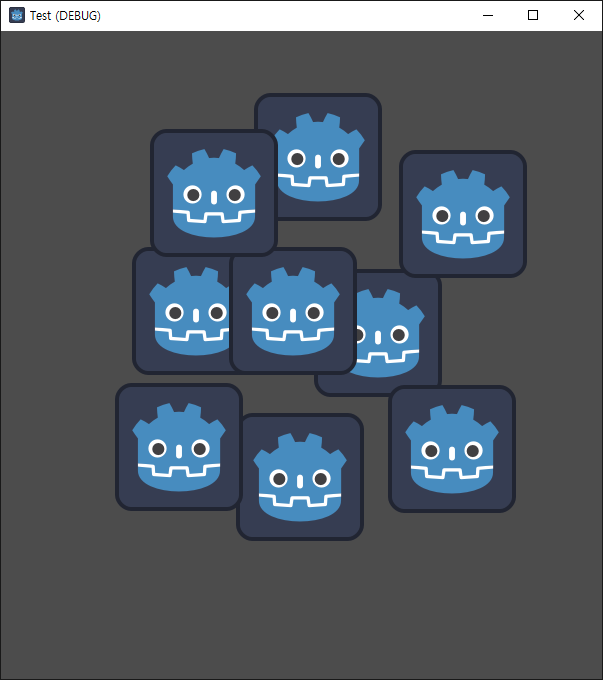
※ 참고
반응형
'Godot' 카테고리의 다른 글
[Godot] Character Move 캐릭터 이동 (0) | 2023.09.20 |
---|---|
[Godot] Keyboard, Mouse Input Handling 키보드 마우스 입력 (0) | 2023.09.20 |
[Godot] Asynchronously Wait 비동기 대기 (0) | 2023.09.19 |
[Godot] Using Custom Signals 사용자 시그널 사용하기 (0) | 2023.09.18 |
[Godot] Using Timer Signal 타이머 시그널 사용하기 (0) | 2023.09.18 |